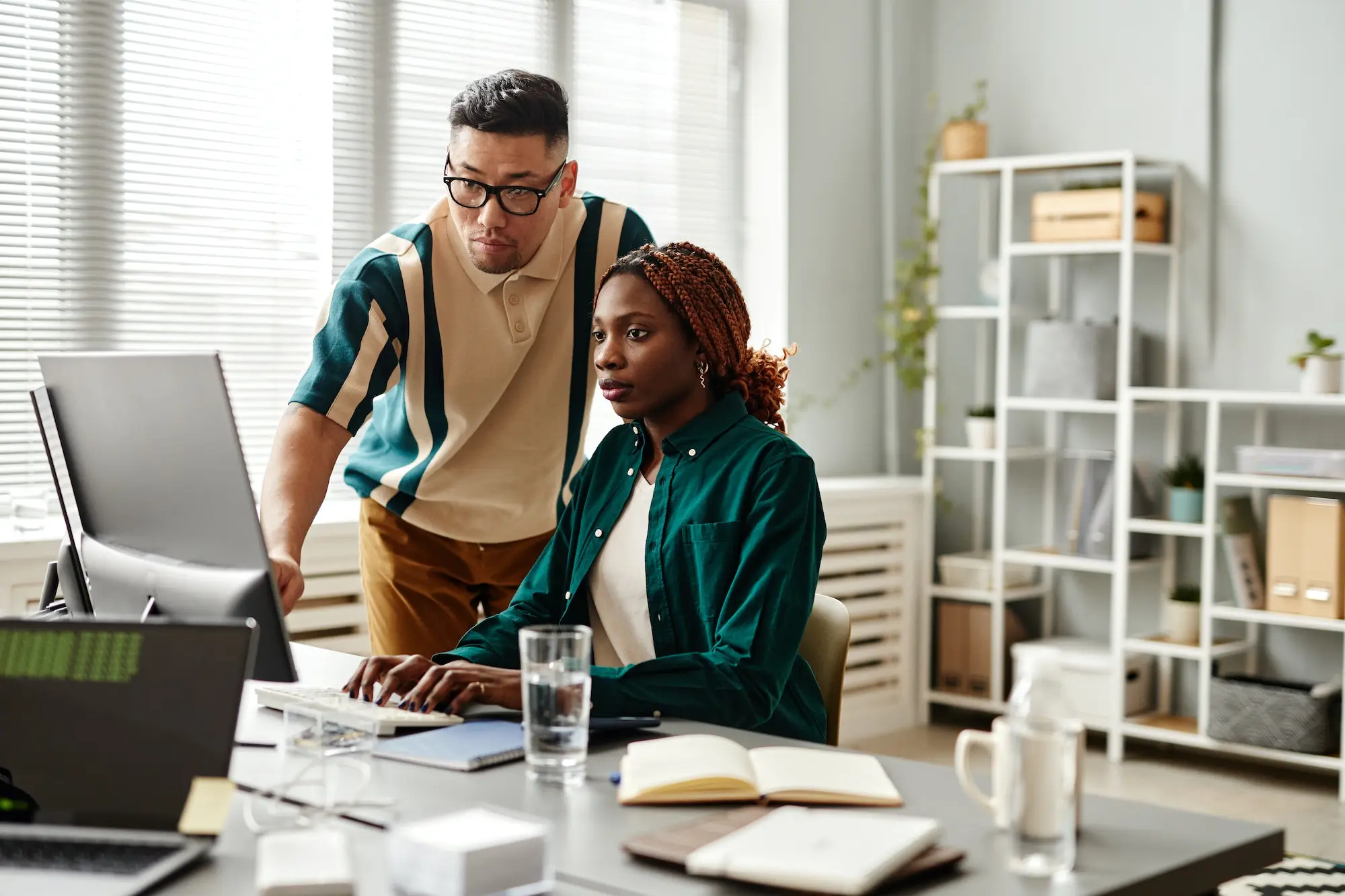
Making sure the process flow of your application is 100% as intended with no room for error. Even making a one-liner change can prompt most developers into doing 3 different tests, just to make sure the code is working as intended.
In this article I am going to delve deep into how we as software developers continuously test and iterate through the code we have written. No matter if you’re working on the front-end section of the application or the back-end section or even just writing a script to update or retrieve some data, some form of testing is recommended to maintain the safety and integrity of the application. You don’t want your code to be the reason why the long-awaited deployment is delayed while the team is on a tight deadline.
This article will be divided into the following sections, covering a couple of important features that will be useful through the course of software development and testing:
- Breakpoints
- SQL Testing
- Postman
- Using inspect on your browser
Breakpoints
If you are writing code, you will need to learn how to use breakpoints. They are one of the best tools in a developer’s arsenal to debug and test an application. If you are unaware of what a breakpoint is, it is essentially a pause button you can add on multiple lines within your application. Just before the code block that is highlighted by the breakpoint is executed, the application will go into pause mode, which will give you, the developer, a chance to view the data that is being passed to the block of code that you are testing.
Another useful thing that can be done with breakpoints are, when the program is in a paused state after hitting a breakpoint, you can write updates to the class/function, hit save + hot reload and then the updated code will be live for testing when the execution process continues. You can then rewind the execution process by dragging the yellow breakpoint up to a previous line of code. The execution will then resume at that point when the continue button is clicked, giving you the opportunity to retest your block of code with the newly written updates without having to restart the application. Just note this only works when the changes don’t break the application or are changes to the application structure. Making changes to model classes being used to populate data or anything that is not basically data manipulation will require you to rebuild the application.
Another useful trick to take note of is that while the execution process is in pause mode, you can manually update the values of variables to see what would happen if unplanned values were sent through to your block of code. This is a great way of preventing potential bugs that might show up later down the line as you will have already tested against them.
SQL Testing
Have you ever feared executing a script on a table or possibly a couple of tables? Just thinking of what could go wrong if you accidentally update the wrong values or overwrite a data entry that was not intended. Don’t worry, with transactions you don’t have to fear messing up.
Testing your scripts in a transaction with a rollback at the end is one of the smartest choices you can make while executing SQL statements. Of course, it is not needed when using select statements, but it will always be recommended when inserting, updating, or deleting data, even making changes to a table’s structure, always put you script inside of a rollback transaction.
This will give you the perfect testing environment where you can try different approaches to solve the problem at hand without any fear. As a matter of fact, this does not only take away your fear, but drastically increases the speed at which you work. If you know that the code is safely tucked away in a rollback, then pressing that execute buttons happens all so very often.
With the knowledge of how to safely write SQL statements, lets now look at the statements itself. In some cases, you will be asked to write complex update or insert statements, even delete statements, that involves multiple tables that will need to get joined together. When this happens, my advice would be to first start with a select statement, complete your joins, and test your where clause. Only when you are certain you have selected the correct data entries for a that need to get updated or deleted, then you change the select statement into the appropriate one you need. Viewing data while developing will always be better than blind scripting.
Postman
Postman is probably one of the most used tools in my arsenal as a software developer. There are so many things that you can do with Postman to make your life easier. It is generally used to make API calls, mostly to the applications that you are developing, but also to live end points that may provide you with needed data.
Postman allows you to create collections and store any/all API calls that you perform on the platform. You can then also share those collection with other people, either by sharing a workspace or extracting it as files. This is a must for development. A developer always needs a way to make calls to the application that he/she is developing. This also increases overall development time, because only one person can write the call and share it with the rest of the team.
When developing applications that make use of API calls to other application end points, it is advisable to use postman to test and structure the API call first, before trying to write it into your code. Having a working example that contains the correct URL, query parameters and request body, makes it a lot easier and faster to code the call into your application.
Most software applications, if not all, will have multiple environments that you will jump between while iterating through the development of a piece of software. Postman gives you the ability to store multiple environment configuration values. This is in fact extremely useful as you can set any/all environment variables that you will ever need, allowing you to make the same API call from your collection to multiple environments by simply changing the intended environment from a drop-down list and then clicking send. These environment variables can be used in your request to make it more dynamic.
Lastly postman is also useful for structuring data in a readable fashion thanks to its Beautify feature. This is great for quickly rendering your JSON for inspection. See, you don’t even have to make any form of API calls for a reason to start using this magnificent tool.
Using Inspect
For the people developing browser applications, I do hope you know how to inspect a web page. If not, then I would strongly recommend you start inspecting. It is very easy to access, you can either right click on the page you want to inspect, and press Inspect, or you can press F12 if you are using Chrome.
In here, you can manually change any of the html elements or styling that are responsible for how the page looks, which helps a lot when you want to figure out how to better present what is on the page, without the need of having to open your IDE. You also have access to any front-end application logs in the console, which also helps a lot in testing what values are being sent through when you interact with the page.
The previous points should be enough reason to start using the inspect feature, but let’s add some more. You can also access the network tab where all requests will be shown. Sources gives you access to your public files and if you go to Application, there you can view information of cookies and local storage, which is also something that makes life easier when you are testing the flow of the application.
Conclusion
So, to break this down, you can essentially pause and stop the process flow at any given point, giving you access to the data being carried around in the system, without having to log anything in your console. You get a very safe testing and scripting environment, where you can grind out your final script, with the use of transactions. Postman has everything you would need to connect and interact with what you are developing, and having the ability to inspect what is happening on your browser, even being able to make small visual changes, makes life so much easier
As you might have noticed by this point, these principles leave you well equipped for your developing experience, they will get used throughout your entire working day. These are essential for software development as they are smart ways of going through the development process.
Why be slow when you can be smart and get stuff done. Happy coding!